The ElementTraversal interface defines methods allowing to access from one element to another one in the document tree. In conforming implementations of Element Traversal, all objects that implement Element must also implement the ElementTraversal interface.
In this article we will demonstrate how to use ElementTraversal interface. This interface is a set of read-only properties, which allow an author to navigate between elements.
Name | Description |
---|---|
ChildElementCount | Returns the current number of element nodes that are children of this element. |
FirstElementChild |
Returns the first child element node of this element.
|
LastElementChild |
Returns the last child element node of this element.
|
NextElementSibling |
Returns the next sibling element node of this element.
|
PreviousElementSibling |
Returns the previous sibling element node of this element.
|
We will use a demo page with such content:
<div id="demo01"> <h1>Horses for sale</h1> <section> <h2>Mares</h2> <article> <h3>Pink Diva</h3> <p>Pink Diva has given birth to three Grand National winners.</p> </article> <article> <h3>Ring a Rosies</h3> <p>Ring a Rosies has won the Derby three times.</p> </article> <article> <h3>Chelsea’s Fancy</h3> <p>Chelsea’s Fancy has given birth to three Gold Cup winners.</p> </article> </section> <section> <h2>Stallions</h2> <article> <h3>Korah’s Fury</h3> <p>Korah’s Fury has fathered three champion race horses.</p> </article> <article> <h3>Sea Pioneer</h3> <p>Sea Pioneer has won The Oaks three times.</p> </article> <article> <h3>Brown Biscuit</h3> <p>Brown Biscuit has fathered nothing of any note.</p> </article> </section> <p>All our horses come with full paperwork and a family tree.</p> </div> <div> <ul id="demo02"> <li>List <span>item 1</span></li> <li>List item 2</li> <li>List item 3</li> </ul> </div>
The first div-container will be used for demostration of the element outline. Assume, we need to show document structure with indents. To do this we can use FirstElementChild and NextElementSibling properties. The code fragment below shows a method to print document structure starting with root element.
private static void PrintOutlineDemo01(Element element,int level=0) { Console.WriteLine("{0}{1}", Tabs(level), element.TagName); for (var elem = element.FirstElementChild; elem != null; elem = elem.NextElementSibling) { PrintOutlineDemo01(elem, level + 1); } }
If we need to print to print document structure without root, we can go the another way:
private static void PrintOutlineDemo02(Element element, int level=0) { if (element.FirstElementChild == null) return; for (var elem = element.FirstElementChild; elem != null; elem = elem.NextElementSibling) { Console.WriteLine("{0}{1}", Tabs(level), elem.TagName); PrintOutlineDemo02(elem, level + 1); } }
In both examples we used method Tabs to generate tab sequence.
private static string Tabs(int n) { return new string('\t', n); }
If we apply our methods to div-container with id "demo01" in demo page, then we get such results:
PrintOutlineDemo01(_document.GetElementById("demo01"));
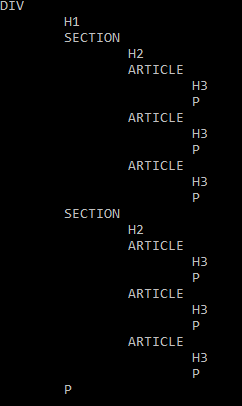
PrintOutlineDemo02(_document.GetElementById("demo01"));
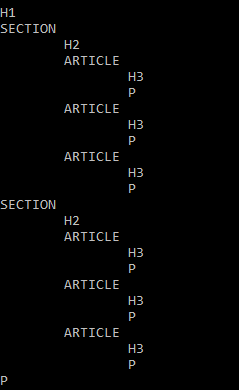
The next example demostated using FirstElementChild, LastElementChild and ChildElementCount properties:
var demoElement2 = _document.GetElementById("demo02"); Console.WriteLine($"Element with id={demoElement2.Id} contains {demoElement2.ChildElementCount} children."); Console.WriteLine($"First child is {demoElement2.FirstElementChild.TagName} with content {demoElement2.FirstElementChild.TextContent}"); Console.WriteLine($"Last child is {demoElement2.LastElementChild.TagName} with content {demoElement2.LastElementChild.TextContent}");
After execution we can see such results:
Element with id=demo02 contains 3 children.
First child is LI with content List item 1
Last child is LI with content List item 3