Introduction
In this article, we will show how you can create a HTML5 document with various CSS styles.
To add CSS styles to your website, you can use three different ways to insert the CSS. We can use an "External Stylesheet", an "Internal Stylesheet", or in " Inline Style". The benefit for using each depends on what you are doing with the Style. The following table explains the difference between them.
Kind | Description |
---|---|
Internal Stylesheet | An internal stylesheet holds the CSS code for the webpage in the head section of the file. This makes it easy to apply styles like classes or ids to reuse The code. The downside of using an internal stylesheet is that changes to the internal stylesheet only effect the page the code is inserted into. |
External Stylesheet | The External Stylesheet is a .css file that you link your website to. This makes it so that what ever you change in the .css sheet, will effect every page in your website. This prevents you from having to make many code changes in each page. This is for "global" site changes. |
Inline Styles | The Inline style is specific to the tag itself. The inline style uses the HTML "style" attribute to style a specific tag. This is not recommended, as every CSS change has to be made in every tag that has the inline style applied to it. The Inline style is good for one an individual CSS change tdat you do not use repeatedly through the site. |
Create the basic HTML5 document from scratch
Let's create the basic HTML5 document as show below.
In this code fragment we use methods
- CreateDocumentType to create a DOCTYPE node
- CreateElement to create appropriate elements
var document = new HTMLDocument();
document.InsertBefore(document.CreateDocumentType("html", "", "", ""), document.DocumentElement);
document.DocumentElement.SetAttribute("lang", "en");
document.Title = "Demo page with CSS style";
document.AppendChild(document.CreateElement("body"));
var head = document.GetElementsByTagName("head")[0];
var metaCharSet = (HTMLMetaElement)document.CreateElement("meta");
metaCharSet.SetAttribute("charset", "UTF-8");
var metaViewPort = (HTMLMetaElement)document.CreateElement("meta");
metaViewPort.Name = "viewport";
metaViewPort.Content = "width=device-width, initial-scale=1";
var linkElement = (HTMLLinkElement)document.CreateElement("link");
linkElement.Href = "http://cdn.myunv.com/css/uecore.css";
linkElement.Rel = "stylesheet";
head.AppendChild(metaCharSet);
head.AppendChild(metaViewPort);
head.AppendChild(linkElement);
In this example, we used the linkElement to create and insert into head-element a following string:
<link href="http://cdn.myunv.com/css/uecore.css" rel="stylesheet">
. As
you can be seen, it's appending an external stylesheet from file style.css. Thus, all styles defined in this
file will be applied to our document.
Add the internal stylesheet to the document
We continue work with our HTML5 document and try to add the internal stylesheet. Similar to the previous example, we create a style-element.
var style = (HTMLStyleElement)document.CreateElement("style");
style.TextContent = "h1 {color: red;} h2 {color:green}";
head.AppendChild(style);
To save a results we will write a simple method WriteSnapShot to get a snapshot of our page. For brevity, we don't discuss this here.
private static void WriteSnapShot(Document document, string filename)
{
using (var device = new ImageDevice(new ImageRenderingOptions
{
Format = ImageFormat.Png
}, filename))
using (var renderer = new HtmlRenderer())
{
renderer.Render(device, document);
}
}
We will also create several headings and paragraphs to display the results of our actions.
var header1 = (HTMLHeadingElement)document.CreateElement("h1");
header1.TextContent = "Heading 1";
var header2 = (HTMLHeadingElement)document.CreateElement("h2");
header2.TextContent = "Heading 2";
document.DocumentElement.LastElementChild.AppendChild(header1);
document.DocumentElement.LastElementChild.AppendChild(header2);
// TODO: Add other demos here
var tempFileName = Path.GetTempFileName();
Console.WriteLine($"Writing snapshot to file {tempFileName}");
WriteSnapShot(document, tempFileName);
At this point we'll get a snapshot like this:
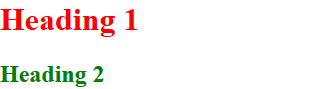
Next, add 10 paragraphs with different text colors, weight and alignment. To generate the text, we will use the Lorem.NET package.
var colors = new[] { "red", "orange", "black", "green", "blue", "indigo", "violet" };
var alignment = new [] {"left", "right", "center"};
for (var i = 0; i < 10; i++)
{
var paragraph = (HTMLParagraphElement)document.CreateElement("p");
paragraph.Id = $"par{i}";
paragraph.SetAttribute("style", $"font-weight:{(i % 2 == 0 ? 700 : 400)}; color:{colors[i % 7]}; text-align:{alignment[i%3]}");
paragraph.TextContent = LoremNET.Lorem.Paragraph(3, 3);
document.DocumentElement.LastElementChild.AppendChild(paragraph);
}
Console.WriteLine(document.Doctype);
Console.WriteLine(document.DocumentElement.OuterHTML);
Now we'll get a snapshot like this:
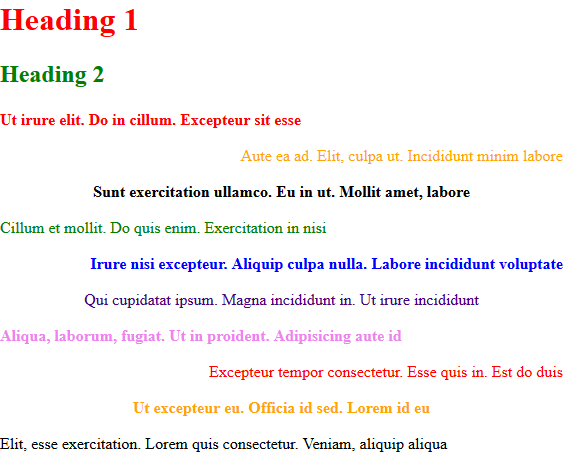
As you can be seen, we used a SetAttribute method to apply CSS rules to element. There are also other ways for setting styles.
All descendants of HTMLElement have a Style property, so, another way to set styles is use this property. Setting a CSSTest attribute of Style will result in the parsing of the new value and resetting of all the properties in the declaration block including the removal or addition of properties.
for (var i = 0; i < 10; i++)
{
var paragraph = (HTMLParagraphElement)document.CreateElement("p");
paragraph.Id = $"par{i}";
paragraph.Style.CSSText = $"font-weight:{(i % 2 == 0 ? 700 : 400)}; color:{colors[i % 7]}; text-align:{alignment[i % 3]}";
paragraph.TextContent = LoremNET.Lorem.Paragraph(3, 3);
document.DocumentElement.LastElementChild.AppendChild(paragraph);
}
Yet another way is to use SetProperty method. This method used to set a property value and priority within this declaration block.
for (var i = 0; i < 10; i++)
{
var paragraph = (HTMLParagraphElement)document.CreateElement("p");
paragraph.Id = $"par{i}";
paragraph.Style.SetProperty("font-weight", i % 2 == 0 ? "700" : "400","");
paragraph.Style.SetProperty("color",colors[i % 7],"");
paragraph.Style.SetProperty("text-align", alignment[i % 3], "");
paragraph.TextContent = LoremNET.Lorem.Paragraph(3, 3);
document.DocumentElement.LastElementChild.AppendChild(paragraph);
}